本文最后更新于 2024年4月4日 下午
LeetCode 232 用栈实现队列 (JavaScript版😎)
题目描述
使用栈实现队列的下列操作:
push(x) – 将一个元素放入队列的尾部。
pop() – 从队列首部移除元素。
peek() – 返回队列首部的元素。
iSEmpty() – 返回队列是否为空。
说明:
你只能使用标准的栈操作 – 也就是只有 push to top, peek/pop from top, size, 和 is empty 操作是合法的。
示例:
1 2 3 4 5 6
| const queue = new MyQueue(); queue.push(1); queue.push(2); queue.peek(); queue.pop(); queue.empty();
|
实现过程
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
|
function MyQueue(contents = []) { this.stack = [...contents]; this.tempStack = []; }
MyQueue.prototype.push = function (x) { this.stack.push(x); }
MyQueue.prototype.pop = function () { let res; while (this.stack.length > 0) { this.tempStack.push(this.stack.pop()); } res = this.tempStack.pop();
while (this.tempStack.length > 0) { this.stack.push(this.tempStack.pop()); } return res; }
MyQueue.prototype.peek = function () { return this.stack[0]; }
MyQueue.prototype.isEmpty = function () { return !this.stack.length; }
MyQueue.prototype.size = function () { return this.stack.length; }
|
ES6 class 实现:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| class MyQueue { constructor(contents = []) { this.stack = [...contents]; this.tempStack = []; } push(x) { this.stack.push(x); } pop() { let res; while (this.stack.length > 0) { this.tempStack.push(this.stack.pop()); } res = this.tempStack.pop(); while (this.tempStack.length > 0) { this.stack.push(this.tempStack.pop()); } return res; } peek() { return this.stack[0]; } isEmpty() { return !this.stack.length; } size() { return this.stack.length; } }
|
运行结果
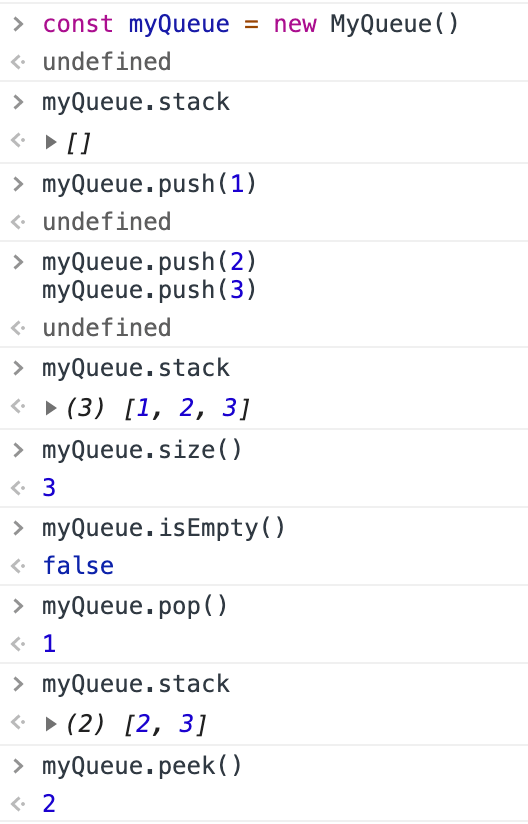